In this article, you'll learn about C++ statements: break and continue. Mores specifically, what are they, when to use them and how to use them efficiently.
In the above program, the test expression is always true.
The user is asked to enter a number which is stored in the variable number. If the user enters any number other than 0, the number is added to sum and stored to it.
Again, the user is asked to enter another number. When user enters 0, the test expression inside
if
statement is false and body of else
is executed which terminates the loop.
Finally, the sum is displayed.
C++ continue Statement
It is sometimes necessary to skip a certain test condition within a loop. In such case,
continue;
statement is used in C++ programming.Syntax of continue
continue;
In practice,
continue;
statement is almost always used inside a conditional statement.Working of continue Statement
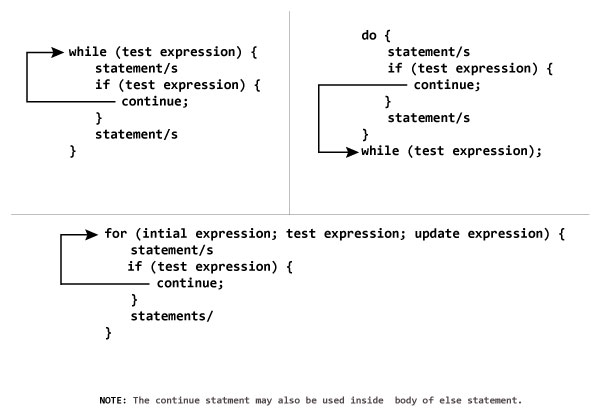
Example 2: C++ continue
C++ program to display integer from 1 to 10 except 6 and 9.
#include <iostream>
using namespace std;
int main()
{
for (int i = 1; i <= 10; ++i)
{
if ( i == 6 || i == 9)
{
continue;
}
cout << i << "\t";
}
return 0;
}
Output
1 2 3 4 5 7 8 10
In above program, when i is 6 or 9, execution of statement
cout << i << "\t";
is skipped inside the loop using continue;
statement.