Public, Protected and Private Inheritance in C++ Programming
In this article, you'll learn to use public, protected and private inheritance in C++. You'll learn where and how it is used, with examples.
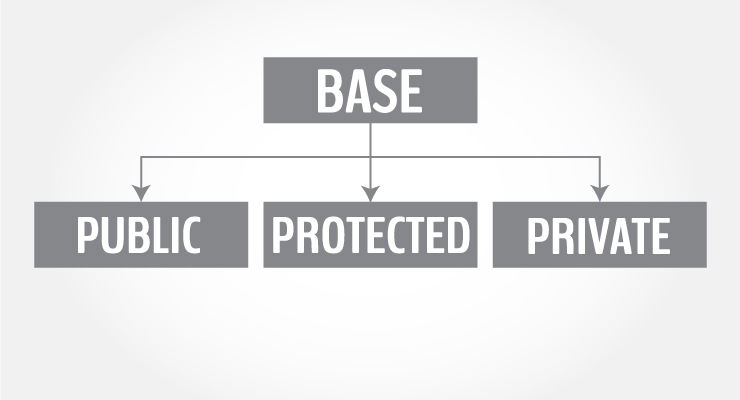
You can declare a derived class from a base class with different access control, i.e., public inheritance, protected inheritance or private inheritance.
#include <iostream>
using namespace std;
class base
{
.... ... ....
};
class derived : access_specifier base
{
.... ... ....
};
Note: Either
public,
protected or
private keyword is used in place of
access_specifierterm used in the above code.
Example of public, protected and private inheritance in C++
class base
{
public:
int x;
protected:
int y;
private:
int z;
};
class publicDerived: public base
{
// x is public
// y is protected
// z is not accessible from publicDerived
};
class protectedDerived: protected base
{
// x is protected
// y is protected
// z is not accessible from protectedDerived
};
class privateDerived: private base
{
// x is private
// y is private
// z is not accessible from privateDerived
}
In the above example, we observe the following things:
base
has three member variables: x, y and z which are public
, protected
and private
member respectively.
publicDerived
inherits variables x and y as public and protected. z is not inherited as it is a private member variable of base.
protectedDerived
inherits variables x and y. Both variables become protected. z is not inherited
If we derive a class derivedFromProtectedDerived
from protectedDerived, variables x and y are also inherited to the derived class.
privateDerived
inherits variables x and y. Both variables become private. z is not inherited
If we derive a class derivedFromPrivateDerived
from privateDerived, variables x and y are not inherited because they are private variables of privateDerived.
Accessibility in Public Inheritance
Accessibility |
private variables |
protected variables |
public variables |
Accessible from own class? |
yes |
yes |
yes |
Accessible from derived class? |
no |
yes |
yes |
Accessible from 2nd derived class? |
no |
yes |
yes |
Accessibility in Protected Inheritance
Accessibility |
private variables |
protected variables |
public variables |
Accessible from own class? |
yes |
yes |
yes |
Accessible from derived class? |
no |
yes |
yes
(inherited as protected variables) |
Accessible from 2nd derived class? |
no |
yes |
yes |
Accessibility in Private Inheritance
Accessibility |
private variables |
protected variables |
public variables |
Accessible from own class? |
yes |
yes |
yes |
Accessible from derived class? |
no |
yes
(inherited as private variables) |
yes
(inherited as private variables) |
Accessible from 2nd derived class? |
no |
no |
no |